What I love and hate about JavaScript
JavaScript is a dynamically typed language, and that gives it a lot of edge in my opinion. It is one of the most used programming language right now. So definitely worth learning even if people do not want to head towards this direction in their careers.
I really enjoyed coding in JavaScript when I first started working with it. Some languages such as Java can be dry and boring to learn, due to the strictness of its syntax. For a new learner this reduces work efficiency, and makes the coding experience less enjoyable. With JavaScript it is a different story, the language is flexible and allow programmers to code the logic of their applications without having to be constantly interrupted by errors messages.
Using undeclared variable in JavaScript is allowed and everything will work just fine. Whereas other languages such as Java will throw about hundreds of errors (okay, I might have exaggerated) when compiled. Here's an example with undeclared variables.
y = 8;
x = 5;
z = y + x; // this will give you 13
This is amazing, because when I am trying to code something there's no need to think about the type or memory allocation when compared to languages such as C. It might not seem like a big thing, but it takes an extra step from the process of converting your thoughts into code.
Now, on to the problems. Even for experienced developer it is still a terrible idea to code like this. The lack of strictness makes it very hard for people to understand the code. JavaScript does some weird interpretations of undeclared variables.
If there is a bug, it will be very difficult to spot even for the person who wrote the code. It doesn't take too many badly managed code base to convince someone to write good code. Let's go through some examples.
- Undeclared variable within a function becomes a global variable:
// can use x here outside of the function
function foo() {
x = 5;
}
- Look at this stackoverflow question and see how confusing it is to just check if a variable has been set
- Types are not what you expect
var x = "Hello";
var y = new String("Hello");
(x === y) // is false because x is a string and y is an object
- Type conversion when you don't expect it
var x = "Hello"; // x is a string
x = 5; // now x is an integer
If the above is not enough to convince you dynamic type is not enough there are plenty of other examples on the web. In general this makes large web applications extremely unstable and difficult to maintain. Through experience I think everyone will slowly feel the pain. I was such a strong supporter of dynamic languages, but looking at code bases with terrible code quality makes it really hard to appreciate the benefits it brings.
Luckily, we can stop this by doing the following:
- Using
'use strict';
within functions/scripts, this will enforce some strict nature in the code - Follow a JavaScript Style Guide within your team to enforce the coding standard
- Using a JavaScript Linter with your IDE or Text-editor
- Add a linter check in the CI process as well to doubly ensure code quality
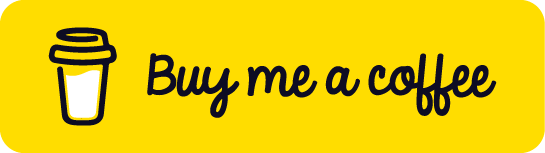