Web Application Rapid Prototyping
First of all, I just want to say that I love the concept of rapid prototyping!
Why? There are so many reasons, but here are just a few which I value deeply:
- Different to normal development - It gives you a whole new perspective to software development, normal expectations and assumptions are not valid here. Testing? Hmmm, maybe later. Team chat about coding standard? No need, we will use someone else's lint rules.
- Learning opportunities - It really tests your knowledge of different toolsets, libraries, and services. If you are working with other people, then there is a high chance of you picking up something you didn't know before.
- Achievement - Literally build something from nothing in a matter of hours or days.
It might not get talked about a lot, but the use cases are probably more common than you think. It could be creating a proof of concept for a client about a potential project, doing a 24h or 48h hackathon or just get out some crazy personal projects like "implement the next Facebook app over a weekend". Either way, it takes a great number of trial and error before it's possible to narrow down to a set of tools and libraries which will ensure the "rapid" part of prototyping. In this blog, I'll be sharing my favourite tools and set up for such scenarios.
What it means to be rapid?
To be rapid, we need to cut down as much work as possible (duh!). This means anything that has nothing to do with building the actual product (look, feel and functionality) is dead weight. Even with the aforementioned three things, if we can avoid reinventing the wheel when we should. Here are some things to consider:
- Don't spend ages setting up a custom webpack build script, project configs or install NPM dependencies manually. Use a CLI or something similar to generate a workable project in minutes, then build the app and deliver value. Don't be afraid of standing on the shoulders of giants!
- Use a UI library instead of writing custom styling, or take it to the next level using a component library. Which will even save you time from adding styling to components such as buttons and form elements.
- Don't build your own CI/CD pipeline, often it takes time to put together or configure. Time is too precious to be used for these kinds of activities, use an off-the-shelf product. For prototypes, I think 99% of the time they will be more than enough.
- Don't build custom APIs, and don't spin up your own Databases. There is also the off-the-shelf solution that are simply too brilliant to ignore.
- At this stage often there aren't enough people working on the same codebase, so there should not be a lot of friction. Keep the releases regular and iterate quickly. I recommend using the Github flow for any projects.
Then the interesting question is since there are so many libraries, tools and SaaS(s?), what is the easiest, fastest and most scalable way to create a web app? That is not only easy to set up but also easy to iterate on top of and scale up (if we hit the jackpot!) to be able to serve to more users. Some may question why scalability is a concern here, I would return the question and ask "what if you can get it with this set up for free?" :)
Steps we can't bypass
No matter how much we simplify the process there will always be a few areas we cannot remove completely. These are:
- Creating the application - implementing features that differentiates itself from other products on the market, otherwise, there is no need of creating this prototype right?
- Production environment setup - the code must be hosted somewhere in one form or another. So that when the user enters an URL they can visit the application.
- Release new changes - there must be a way to have one version on the production environment, whilst developing new features. Then when the new feature is ready, release the new feature to the production environment.
- Dynamic functionalities - be able to save data and provide a complex and dynamic user experience, most likely through using external APIs and SaaS.
We will cover each of the above in more depth in the following sections.
Easiest way to creating the application
The simplest way to creating web apps is using something like create-react-app
repo as a starting point or simply generate a get started project using Vue CLI
. I am not an Angular developer, but there is also the Angular CLI
tool as well.
In this case, I would use something like Vue CLI
. I won't cover too much detail here, because I've already covered it in another blog post. It's a simple 10 steps even if you want to configure everything yourself.
If getting the ideas out there is a top priority, then even consider not including things such as E2E testing. Although, I'd recommend against that if there's a chance of building on top of this in the future. Maybe the best option is to include it in the setup but not add any test until a decision has been made, as it is always easier to remove than to add later.
I have deliberately left out UI and component libraries because there are so many out there, so just pick one that works best for you.
App Hosting
Developers no longer need to pay for app hosting. There are so many free or free-tier services on the market now, so don't waste money on this.
There are a few that comes to mind, but the one I recommend is Netlify. Check out the link for more info, but just to mention a few key features:
- Automate build & deployment based on webhook
- Each push to preview your changes and a Netlify URL for all your branches
- Built-in SSL/TLS and certification (Let's Encrypt)
- And many more
The setup process is also very straight forward. Login with Github, then select the repository you'd like to set up.
Enter in the build command (e.g. npm run build) and folder where the app is generated (e.g. dist folder). Voila!
Of course, there are lot more settings for you to custom (like previously mentioned SSL/TLS certificates, custom domain and etc), but this is all it takes to get everything started.
Release new versions
Not much to say here. With Netlify, it automatically creates webhook with Github so that when the code is pushed (to any branch) it could trigger a build. This means when you want to merge a PR, it will also tell you if the code is working correctly.
Dynamic functionalities
With any web application, we will need to use APIs or other means to communicate with a database in order to read and write user data. This is to ensure when the user return, they can resume from where they left off. In other cases, it could be used to provide the user information to improve their experience. E.g. typeahead with a list of countries in a country form field instead of asking the user typing it in manually.
The service I like the most is Google's Firebase. It has so much features baked in, things like OAuth, sign up, forget password and etc. But most importantly, its real-time database. This will allow us to build the application that can change on user's device in real time (hence the name real-time database). For example, if I have a chat app open on two devices. If I send a message on one of these devices then the other device will simultaneously show up without the user having to refresh the page or interact with the app. This is seriously powerful stuff, these kinds of features used to be a headache to build with socket.io.
The setup process for Firestore is very well documented by Google. Check out the official doc. If you are too lazy to click open the link, then TL;DR is:
- Install NPM dependency
- Initialize Cloud Firestore by adding in API keys and called
firebase.firestore()
in the app
It worth noting the API keys should be version controlled because it will then forever be on Github or other source control tools. I'll cover the best way to set up Firebase in a different blog post, as it is not quite as relevant to rapid prototyping.
Summary
To conclude, the best way to rapidly set up a CI/CD pipeline, codebase and production environment is by using the following tools:
- Vue CLI
- Netlify
- Firebase
Here's a diagram illustrating how everything fits together.
If you run through the above steps a couple of times, I'm sure it's possible to get a prototype project set up in less than 5 mins (excluding the Vue CLI dependencies build time of course).
Alternative tools
As mentioned already, there are simply too many tools out there for me to include in one set up. Most of them have overlapping use cases or is not as powerful as the tools mentioned above. But I am aware depending on the project idea, the requirement for tools can be very different so here are a few other tools which I've been very impressed in the past:
- Contentful - if what you are building requires a CMS, then this is a strong contender in this field. I am personally using this for this blog, and I can say a lot of great things about it.
- Apiary - If you do need to create your own custom API for a project but don't want to actually waste time building it. This is a cool way to mock some responses then build the real ones later.
- Cloudnary - creating an image-heavy app? Definitely check out this service, told simply it hosts media files for you e.g. images and videos. But they allow dynamically change image size, rotations, cropping and etc via URL param or using their SDK.
I tried to keep the explanation short, so check out their websites for more information. They will definitely sell themselves than I ever could :D
Final words
I'm always looking for new cool tools, libraries and services. If you think I've missed something, then I'd love to know! Get hold of me either by leaving comments here or ping me on Twitter.
I hope this article was helpful, thanks for reading!
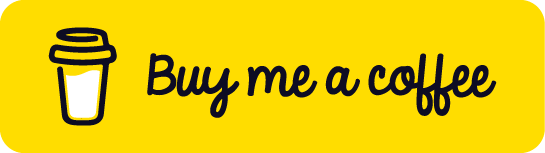