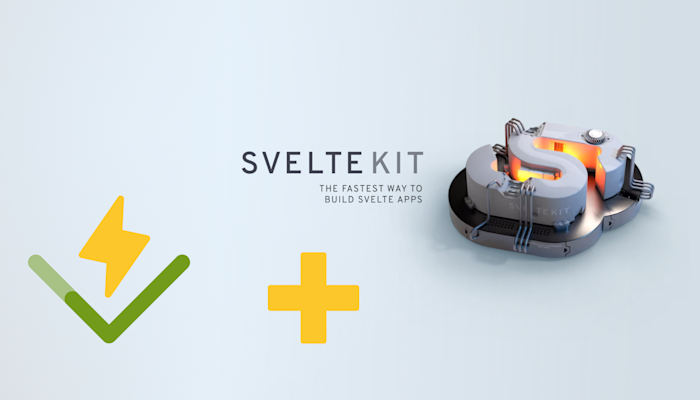
Setting up Vitest within SvelteKit repo
SvelteKit doesn't come with unit test tools out of the box, so we'll have to configure our own. Usually, I'd add in Jest because it's always been my favourite testing framework. However, I recently heard good things about Vitest and thought I'd give it a try. For those unfamiliar with Vitest, it is a drop-in replacement for Jest and is much faster. In addition, I've noticed another benefit of how simple it is to configure compared to Jest since it natively supports TypeScript. However, we still need to do a couple of things to get Vitest working properly with SvelteKit. Let's go through what these things are.
Install Vitest
First, we need to install it. So go ahead with one of the following commands depending on the configuration of your project.
// with npm
npm i -D vitest
// or with yarn
yarn add -D vitest
// or with pnpm
pnpm add -D vitest
If you need more information, maybe follow the official guide.
Configure Vite/Vitest
Once Vitest has been configured, we need to update vite.config.ts to look like the following:
/// <reference types="vitest" />
import { defineConfig } from 'vite';
import { svelte } from '@sveltejs/vite-plugin-svelte';
export default defineConfig({
plugins: [svelte({ hot: !process.env.VITEST })],
test: {
globals: true,
environment: 'happy-dom'
}
});
Notice the /// <reference types="vitest" />
at the top of the file in order to define test inside defineConfig. If we don't add this, we'll get the following error message because the defineConfig type doesn't understand the test
property.
Here's the full error message:
Argument of type '{ plugins: Plugin[]; test: { globals: boolean; environment: string; }; }' is not assignable to parameter of type 'UserConfigExport'.
Object literal may only specify known properties, and 'test' does not exist in type 'UserConfigExport'.ts(2345)
Configure TypeScript
Next, we need to configure TypeScript, so it understands the globals such as describe, test, or it. The tsconfig.json should be just extending the default tsconfig in svelte-kit. We want to add "vitest/globals"
type in compilerOptions.
{
"extends": "./.svelte-kit/tsconfig.json",
"compilerOptions": {
"types": ["vitest/globals"]
}
}
If we don't do this, when you create or open a test file, you'll see the following errors. It is because typescript doesn't know where to look up these globals.
Again, here's the full error message:
Cannot find name 'describe'. Do you need to install type definitions for a test runner? Try `npm i --save-dev @types/jest` or `npm i --save-dev @types/mocha`.ts(2582)
All done
If you make those two small config file changes, everything should work as expected.
I'm having a blast with Vitest, love the improved SPEED! More importantly, coming from Jest, I didn't need to re-learn any new APIs. In fact, I don't even remember I'm working with a different test framework most of the time.
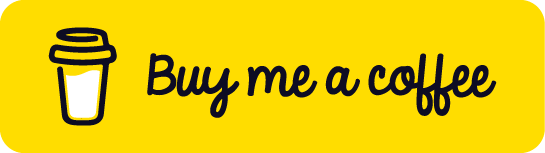