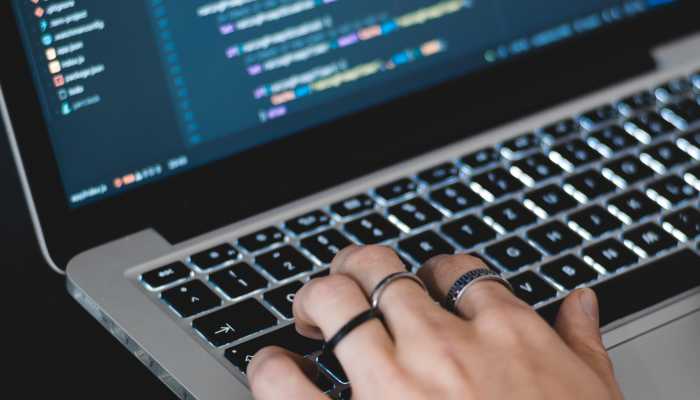
The best programming language to use in coding interviews
As I've mentioned in a previous blog post Becoming an interview engineer, I have been an interview engineer at Karat for about six months now. As an interviewer, it is my job to observe different candidates attempt the same questions. Over time, I noticed something intriguing - the best performing candidates I interviewed all use the same programming language. To make it clear, Karat allows candidates to pick from 26 programming languages (excluding Markdown) when attempting coding interviews. Out of these languages, candidates tend to choose the same few languages. Out of these languages, there's a clear winner when it comes to the best language to use for coding interviews - Python. Of course, candidates can still perform well using other languages, but I strongly feel the interviewing "game" is rigged against them.
The best candidates I've interviewed all used Python 3. I don't think the version of Python matters here, but I thought I'd be accurate. So let's talk about why I think Python is the best language to use in coding interviews (if you are familiar with it already).
Disclaimer: before people assume I'm just a Python fanboy, I want to clarify that it isn't my language of choice, and it isn't something I use regularly. I can write basic scripts in Python, and it wouldn't be hard for me to pick it up again quickly. But I wouldn't put myself even close to the Python camp based on my skills and experience. So hopefully, that proves I'm not being biased here.
Indentation > curly-brace system
As the interviewer, I cannot say anything during interviews that directly helps the candidate, or they will get marked down for my comments. So imagine how painful it is for me to watch people spend a few minutes trying to fix a missing curly bracket.
It might sound stupid to suggest brackets are a big deal. But when interviewing, people tend to make more mistakes due to the time pressure and knowing someone is watching them code. Our brains focus on the interview question and don't pick up simple syntax issues straight away. Coding slowly isn't an option to get the best marks since companies want fast and accurate solutions. When people rush, minor issues like missing brackets become harder to spot. I once watched someone spend 10 minutes struggling with a bracketing issue. Based on comments from other interviewers, I wasn't the only one either.
If you are not convinced, I've included some basic code snippets below to illustrate how the brackets can be confusing when coding under pressure. It is also worth noting that the code block tends to be more complex and be much longer in interviews, which makes spotting missing brackets even harder.
# Python
def helloWorld:
for i in range(len(some)):
for j in range(len(random)):
if falseCondition:
for k in range(len(random)):
...
// JavaScript
function helloWorld() {
for (const i in some) {
for (const j in random) {
if (falseCondition) {
for (const k in loop) {
...
}
}
}
}
}
}
Did you notice there is one too many bracket in the JavaScript code?
Dynamic type = less code = code faster
When it comes to writing code fast, it is tough for statically typed language to complete with dynamically typed languages. Things as basic as declaring a function or variable are faster to do in Python than in a language like Java.
Here is a comparison between declaring a function that takes in a 2D integer array and returns the same type in Python and Java, respectively.
# Python
def myFun(arr):
// Java
public static int[][] myFun(int[][] arr) {
Python developer has already started implementing the function by the time Java developer types out the word "static". Yes, this is just one line of code, but Python code is always shorter with the same approach. A few characters here and there don't sound much. But when there are tens or hundreds of lines of code, it all adds up because it gives a developer more time to be debugging code instead of typing things out.
Longer code also means more likely for mistakes to creep in. For example, people make mistakes with function data types or forget to type the "static" keyword when declaring the function. With dynamically typed languages, you simply don't have these concerns. Even compared to another dynamically typed language like JavaScript, I have to admit Python code is a lot faster to write because it requires fewer characters to implement the same logic.
"Statically typed languages have advantages too!" I hear you say. That's 100% true, and I agree. Personally, I went from extremely against statically typed language at the start of my career to starting every single project now using TypeScript. Unless I'm throwing something together quickly, TypeScript gets my vote every time. Types come with safety and guarantee, and IDE can leverage it to give better autocomplete, it can catch issues that dynamically typed language cannot, and plenty more advantages. But when shorter code beats good code in an interview, dynamically typed languages win hands down. Like many places, at Karat, we put much more emphasis on working solutions than nice-looking code.
Some languages are better for implementing algorithmic questions
Maybe it's because I'm a JavaScript developer, so this is a more significant pain point. For others languages, this might be irrelevant. But, as a JavaScript developer, I find the lack of useful util functions makes coding algorithm solutions significantly harder.
To demonstrate my point further, here is a comparison between Python and JavaScript for finding the intersections between two arrays. It is not just that the Python code is shorter; the JavaScript code is also harder to read and understand. All this adds friction to the interview process.
# Python
def intersection(a, b):
return list(set(a).intersection(b))
// JavaScript
function getIntersection(a, b) {
const set1 = new Set(a);
const set2 = new Set(b);
const intersection = [...set1].filter(
element => set2.has(element)
);
return intersection;
}
Would I find an intersection like this in an interview when coding in JavaScript? Of course not. My first choice will be to implement the solution using forEach()
loop because I'm more familiar with it. In interviews, familiarity is king. But this implementation isn't any quicker to write and could introduce unnecessary bugs than the Python approach. It is also inferior in terms of time complexity.
function getIntersection(a,b) {
const arr = [];
a.forEach(elem => {
if (b.contains(elem)) {
arr.push(elem);
}
}
const arr;
}
To ensure this isn't a problem that only partially affects JavaScript developers, I researched online and found this question on stackoverflow, so it seems GoLang has a similar problem.
Conclusion
Now, should you drop everything and learn Python for all future interviews? No, that would be crazy. People can be good at any language they spend time mastering. The better thing to do is practice with more interview-style coding questions and find a solution faster. Finding the most straightforward solution will save you more time than switching to Python and typing less code. It is often best to go with something more familiar and comfortable than to min-max the benefits I explained above in time-sensitive environments. However, for people who already use multiple languages where Python is already in their toolbox, I'd strongly recommend using it for interviews unless the job requires a specific language.
Some candidates ask me how come I became an interview engineer. It always sounds like a cliche, but I enjoy seeing different people approach the same interview questions from different angles and coming up with unique solutions. Furthermore, I often learn something from the candidates I've interviewed. Believe it or not, an interview I recently conducted inspired this blog post. If this sounds like something you are interested in, you can apply to become an interview engineer using this link (if you are from the US) or this link (from any other country)!
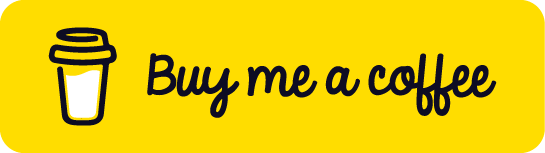