JavaScript Events
We are always using JavaScript events when developing web application, it is what makes it interactive at the end of the day. In this blog, I'll go over what it is and the different ways to intercepted a JavaScript event.
JS events in HTML
HTML events are “things" that happen to HTML elements. We can use JavaScript to “react” to these events. Events can be:
- An HTML web page has finished loading
- An HTML input field was changed
- An HTML button was clicked
Here are some common HTML events:
onchange
- An HTML element has been changedonclick
- The user clicks an HTML elementonmouseover
- The user moves the mouse over an HTML elementonmouseout
- The user moves the mouse away from an HTML elementonkeydown
- The user pushes a keyboard keyunload
- The browser has finished loading the page
<button onclick="displayDate()">The time is?</button>
In summary, this section explained how to use events in HTML elements.
Source: https://www.w3schools.com/js/js_events.asp
JS events in JS
Events is the fundamentals to how modern framework achieves data bindings. Angular 1.x is probably the best example, its two way data binding on the lowest level is events.
How to use it
event = new Event(typeArg, eventInit);
typeArg
is a DOMString representing the name of the event.
evenInit
is optional object type, that can take in the following:
bubbles
- A boolean indicating whether the event bubbles. Default value isfalse
.cancelable
- A boolean indicating whether the event can be canceled. Default value isfalse
.composed
- A boolean indicating whether the event will trigger listeners outside of a shadow root. Default value isfalse
.
// create a look event that bubbles up and cannot be canceled
var evt = new Event("look", {
"bubbles":true,
"cancelable":false
});
document.dispatchEvent(evt);
Intercepting an event
There are times when you want to stop an event from doing what it. My most recent experience was, closing an menu when the user clicks anywhere else on the screen. So the code logic was:
- Click anywhere on the screen and hide menu
- When click on the menu, override the above logic and do not hide menu
So now the question is how do we override an event? There are a number of ways to achieve this, we will try to cover most of them here.
1. Event.preventDefault()
preventDefault()
method tells the user agent that if the event does not get explicitly handled, its default action should not be taken as it normally would be. The event continues to propagate as usual, unless one of its event listeners calls stopPropagation()
or stopImmediatePropagation()
, either of which terminates propagation at once.
Usage: Prevent a user from checking a checkbox.
Tip: If an event is not cancelable
, calling preventDefault()
will throw errors. It is recommended to check on cancelable
before invoking preventDefault()
.
2. Event.stopPropagation()
Prevents further propagation of the current event in the capturing and bubbling phases.
3. Event.stopImmediatePropagation()
Prevents other listeners of the same event from being called. It is the same as event.stopPropagation()
plus preventing other registered event handlers on the same element to be executed. So it does not prevent the default action for an event, such as following a clicked link.
4. Return false
Return false as an event is basically the same as calling both, event.stopPropagation()
and event.preventDefault()
.
Tip: return false does not prevent the event from bubbling up in “normal" (non-jQuery) event handlers but still prevents the default action.
Source: https://developer.mozilla.org/en-US/docs/Web/API/Event/Event
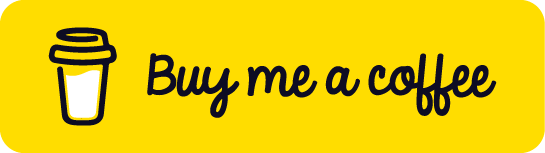